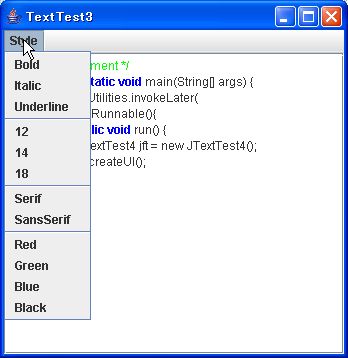
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import javax.swing.Action;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextPane;
import javax.swing.SwingUtilities;
import javax.swing.text.StyledEditorKit;
/**
* @see http://java.sun.com/docs/books/tutorial/uiswing/components/generaltext.html
*/
public class JTextTest4 {
private JFrame frame;
private JTextPane txtPane;
/**
* StyledEditorKit を利用する
*/
private JMenu getMenu() {
JMenu menu = new JMenu("Style");
Action action = null;
action = new StyledEditorKit.BoldAction();
action.putValue(Action.NAME, "Bold");
menu.add(action);
action = new StyledEditorKit.ItalicAction();
action.putValue(Action.NAME, "Italic");
menu.add(action);
action = new StyledEditorKit.UnderlineAction();
action.putValue(Action.NAME, "Underline");
menu.add(action);
menu.addSeparator();
menu.add(new StyledEditorKit.FontSizeAction("12", 12));
menu.add(new StyledEditorKit.FontSizeAction("14", 14));
menu.add(new StyledEditorKit.FontSizeAction("18", 18));
menu.addSeparator();
menu.add(new StyledEditorKit.FontFamilyAction("Serif", "Serif"));
menu.add(new StyledEditorKit.FontFamilyAction("SansSerif", "SansSerif"));
menu.addSeparator();
menu.add(new StyledEditorKit.ForegroundAction("Red", Color.red));
menu.add(new StyledEditorKit.ForegroundAction("Green", Color.green));
menu.add(new StyledEditorKit.ForegroundAction("Blue", Color.blue));
menu.add(new StyledEditorKit.ForegroundAction("Black", Color.black));
return menu;
}
private void createUI() {
frame = new JFrame("TextTest3");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
txtPane = new JTextPane();
txtPane.setPreferredSize(new Dimension(200,100));
JPanel basePanel = new JPanel(new BorderLayout());
basePanel.add(new JScrollPane(txtPane), BorderLayout.CENTER);
// メニューを作成
JMenu menu = getMenu();
JMenuBar mb = new JMenuBar();
mb.add(menu);
frame.setJMenuBar(mb);
frame.getContentPane().add(basePanel);
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(
new Runnable(){
public void run() {
JTextTest4 jft = new JTextTest4();
jft.createUI();
}
}
);
}
}
YAGI Hiroto (piroto@a-net.email.ne.jp)
twitter http://twitter.com/pppiroto
Copyright© 矢木 浩人 All Rights Reserved.